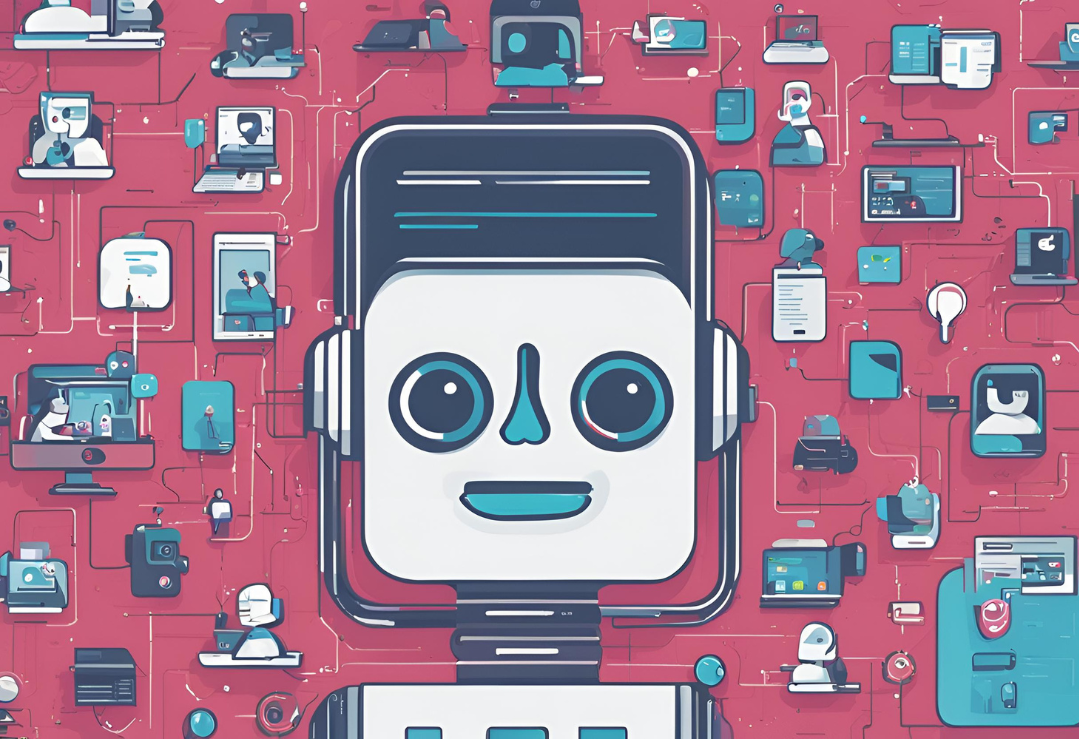
Tutorial: Chatbot in AstroJS with CloudFlare Workers AI (Part 1) - Setting up the Environment
In this tutorial, I will guide you through creating a chatbot using the CloudFlare adapter in an AstroJS project. This first part focuses on setting up a fresh development environment and deploying it to CloudFlare pages. The full code for this tutorial can be found on GitHub.
1. Start a new AstroJS project with Cloudflare adapter
Astro makes starting a new project incredibly easy with a single command that guides you through the process. We’ll use the Cloudflare command to create a new AstroJS project:
npm create cloudflare@latest my-astro-app -- --framework=astro
Replace my-astro-app
with your desired project name. The terminal will prompt you with a few questions about the project. Here’s an example of the answers:
- Ok to proceed? (y): y
- How would you like to start your new project?: Include sample files
- Do you plan to write TypeScript?: Yes
- How strict should TypeScript be?: Strict
- Initialize a new git repository?: Yes
- Do you want to deploy your applications?: No
Once complete, a new directory named my-astro-app
will be created with the necessary project files. Your project structure should resemble the following:
Directorynode_modules/
- …
Directorypublic/
- …
Directorysrc
Directorycomponents/
- …
Directorypages/
- …
- env.d.ts
- …
- astro.config.mjs
- package.json
- package-lock.json
- tsconfig.json
- README.md
- wrangler.toml This file is added by CloudFlare.
The only difference in the file tree compared to a default AstroJS project is the wrangler.toml
file. This file, added by Cloudflare, allows you to incorporate Cloudflare bindings and declare environment variables, which we’ll explore in the next step.
Finally, for deployment to CloudFlare Pages, follow this helpful tutorial
2. Connect your CloudFlare page to CloudFlare Workers AI
You must create an AI binding for your project to connect to CloudFlare Workers AI and use their models. Bindings allow your Workers to interact with resources, like Workers AI, on the Cloudflare Developer Platform.
To bind Workers AI to your Worker, add (or uncomment) the following to the end of your wrangler.toml
file that was created in the previous step:
[ai]binding = "AI"
To ensure proper TypeScript support for Cloudflare Workers AI, you might need to modify your package.json
and src/env.d.ts
files. This step is typically unnecessary if you’ve used the Cloudflare package to initialize your project, as the required build options are automatically added.
If you haven’t, add the following script to your package.json
file under the “scripts” section:
{ "scripts": { "dev": "wrangler types && astro dev", "start": "astro dev", "build": "wrangler types && astro check && astro build", "preview": "wrangler types && astro build && wrangler pages dev", "deploy": "astro build && wrangler pages deploy", "astro": "astro", "cf-typegen": "wrangler types" }}
To access environment variables defined in your wrangler.toml
file from your Astro endpoints and middleware using Astro.locals
, you’ll need to create a Runtime type in your src/env.d.ts
file.
This type will provide TypeScript with necessary type information for your environment variables, ensuring better code completion and type safety.
1type Runtime = import("@astrojs/cloudflare").Runtime<Env>;2
3declare namespace App {4 interface Locals extends Runtime {}5}
With these foundational elements in place, we’re ready to dive into building our chatbot component. The next part of this tutorial will guide you through creating an endpoint to handle incoming chatbot requests.